Load Up the Graphic Files
In Part IV of the Content Management System (CMS) series, I noted that I wanted to have a separate post on uploading graphics to a web server. This post addresses the issues involved in getting graphics in place on a web server that will be included in a Web page, whether you’re using a CMS or not. In the case of selecting a graphic from your desktop and uploading it to a server, most of the important work is done by HTML. So, we’ll start with the HTML assuming that HTML5 is in use. Be advised at the outset that you will need to do a little system administration to set your file privileges in the folders where you will store the graphic files. In the development process, I used a local host, a different computer on my LAN, and two different web servers at remote locations.
Choosing a File to Upload
HTML5 (and possibly earlier versions) has some very nice built-in form attributes that make selecting a graphic file from your computer to place in an “uploadable” state quite easy. All you need are a couple of lines of HTML. Within a form container, the line:
generates the following button when you load the code into a browser:
In the tag, you will need to include an enctype assignment:
That’s about it, and you’re all set to select a file that can be accessed through the PHP superglobal variable $_FILES.
The following HTML code generates the CSS first the then HTML portion of the little graphic CMS:
@charset "UTF-8"; /* CSS Document */ /* upload.css */ body { font-family: Verdana, helvetica, arial, futura, sans-serif; color:#991C00; background-color:#FFFCDB; } h1 { font-family: "Arial Black", futura, sans-serif; color:#E09A25; background-color:#262B30; text-align: center; } |
< !doctype html> |
I added separation between the Choose File button and Load Graphic File submit button so that the HTML and PHP portions are more clearly defined. Figure 1 shows the initial web page for the upload:
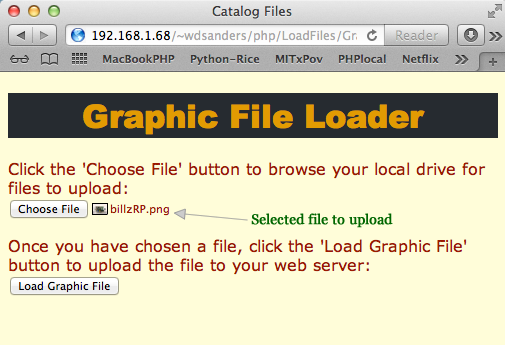
Figure 1: Selecting and calling PHP Script
Since the goal of having a CMS is to make updates simple, this initial example does not have any whistles and bells (e.g., folder selection), and it does nothing more than to prepare a selected file for upload to a server.
A Little PHP
On the PHP side of the equation, you have only two code elements:
- $_FILES: Superglobal array with selected file information in elements
- move_uploaded_file(): Stores uploaded file (in $_FILES) to in specified server location.
Creating a class, using the minimum code and no error, type or size checking, is quite simple. To keep it even simpler, the following trigger script instantiates the Upload class:
< ?php //triggerUpload.php include_once("Upload.php"); $worker = new Upload(); ?> |
After testing the initial Upload class, we’ll see how it can be enhanced. First, though, take a look at the code:
< ?php //Upload.php class Upload { private $fileNow; public function __construct() { if ($_FILES) { $this->fileNow=$_FILES["file"]["name"]; //The following line does all the work move_uploaded_file($_FILES["file"]["tmp_name"],"images/$this->fileNow"); echo $this->fileNow . " has been uploaded to the images folder:"; } } } ?> |
That simple class does everything you need to upload and store a file in a folder named “images” in the directory where the script is launched. It’s pretty basic, but you should be able to see exactly what is required to upload a file to a web server without having to use an FTP client.
Template Method for Details
Note: Before you get started on this section, download all of the files that are involved:
As you saw, the Upload class is quite easy. However, you will want to make sure that not just any file type is loaded and you’ll want to make sure that you don’t have giant files loaded that may fill up your disk space. (You also want to protect against maliciously uploaded files.) The $_FILES array has information to use for checking type and size. Using the $_FILES array information we can boil the process down to three steps:
- checkSize(); See how big it is and compare to a maximum allowable size.
- checkType(); Add a set of acceptable file types used with a Web page and check to see if the file type is acceptable.
- uploadFile(); If the file type and size are okay, upload the file.
Click below to continue:
Continue reading ‘PHP Graphic CMS Class with Template Method’
Recent Comments