Let’s Build Something!
A lot of the work PHP developers do involves creating the same thing with variations. For example, you may create a Web site with different organizations for desktop displays, tablet screens and mobile views. However, you want the content the same, and while set up differently, you want to have a navigation system, headers, links and all the rest that goes into a Web site. The same is true for working with MySQL, and forms of different kinds. You can create fairly complex objects, but the representations (e.g., desktop, smartphone) are different. The Builder Design pattern is a creational pattern that lets you separate the construction of complex objects from their representations so that the same construction process can create different representations. Before going further, take a look at two different results in the PlayA and PlayB buttons and download the source code:
The Simple Builder
This first example looks at creating a simple Builder pattern with all of the parts laid out and pretty spread out. Two Client objects call two different concrete builders, and while that would have been pretty easy to do with a single Client the purpose of this first example is to clearly show each of the parts. In Part II, you’ll see a more streamlined and functional version of a Builder, but for now, it’s important to see how this thing works. Before going further, take a look at the Builder class diagram in Figure 1:
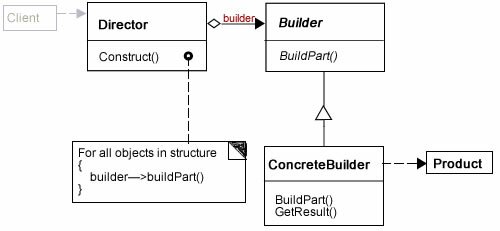
Figure 1: Builder Class Diagram (with Client added)
As noted in Figure 1, I added the Client, and according to the Gang of Four,
The client creates the Director object and configures it with the desired Builder object.
So, the Client, while not a participant in the pattern, is clearly a key collaborator. However, I put the Client code at the end of this post because its role must be understood in the context of the Builder’s key participants.
The Builder Interface
It’s difficult to know where to begin in describing the Builder pattern; but starting with the Interface helps show the main building blocks.
< ?php //IBuilder.php interface IBuilder { public function mainHead(); public function subHead(); public function buildNavH(); public function buildNavV(); public function buildGraphic(); public function buildVideo(); public function buildBodyText(); } ?> |
In looking at the IBuilder interface, you can see what might be the parts of a Web page. Keeping in mind that this example is a simple one, the example is a Web page “wire framer.” That is, it creates a graphic outline of what the page will look like using SVG graphics generated dynamically in the program.
Continue reading ‘PHP Builder Design Pattern Part I: One Process, Many Representations’
Recent Comments