Why ‘Near’ Strategy?
The Strategy design pattern is important because it is so useful, and two different posts on this blog have covered it in the past. What I would like to do with this implementation of the pattern is to do two things: 1) First, show how to create operations that require no conditional statements, and 2) Second, (in Part II) show the role of the Context participant. In this first part, I’d like to look at the HTML->PHP operations where specific objects (classes) can be called through HTML forms.
One of the nice things about the Strategy pattern is that the logic of it is very easy to understand; albeit on a foundational level. You have different tasks, and each task requires a different algorithm; so why not separate the algorithm operations into distinct objects (classes) and loosely bind them to the client? Each class will only do one thing, and that is to carry out an algorithmic operation. To do this, start off with a “Near Strategy”–a pseudo-patten: the Strategy design pattern missing the Context. Figure 1 shows the difference between a Near Strategy and true Strategy design pattern:
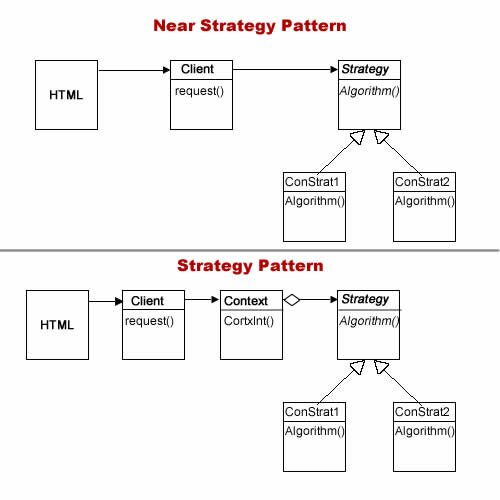
Figure 1: The Near Strategy design and the Strategy Design pattern
The HTML UI and the Client are not a part of the Strategy design pattern, but in this example, both are required for making requests. Before going on, try out the program by clicking the Play button and download all of the files.
You can use the files “as-is” but you will need to change the IConnectInfo.php file so that the hostname (HOST), user name (UNAME), database name (DBNAME) and password (PW) is your actual MySql database. Also, once you create a table with a name of your choosing, be careful not to create it again if you want to keep your data entered into the table!
Using Form Names for Concrete Strategy Names
One of the coolest features of PHP is the ability to use strings to create instances of classes (aka: objects). For example,
$myVar = “MyClass”;
$myObject = new $myVar();
creates an instance of the class, MyClass. Knowing this, we can create the class names in HTML as form values. For instance:
…
…
The selected radio button value can be passed in a super-global to PHP:
…
$myVar = $_POST[‘callme’];
$myObject = new $myVar();
…
Using this, the Client doe not have to work through conditional statements to decide which request to fulfill. Like the Strategy design pattern, even the Client is free from using conditional statements as can be seen in the following listing:
< ?php function __autoload($class_name) { include $class_name . '.php'; } Class Client { private $chooser; private $instance; public function __construct() { $this->chooser=$_POST['sql']; $object=$this->chooser; $this->instance=new $object(); } } $worker=new Client(); ?> |
As you can see the Client class is pretty simple with no conditional statements. It takes the value of the radio button send with a Post method. The radio button group is named “sql”; so whatever button is clicked is translated into an object.
Continue reading ‘PHP Near Strategy Design Pattern Part I: No Conditionals, Please’
Recent Comments