Can We Talk?
The initial discussion of the Memento design pattern illustrated how a state could be saved in a different object than the one in which the state originated. A Caretaker object holds the saved state and when requested, it returns the state to the Originator, all without breaking encapsulation. A practical example of employing the Memento that comes to mind is where the user is looking through a list. As she goes through the list, she sees different items (flowers in this case) that she is considering. However, because it’s a long list, she cannot remember which one she likes; so she tags those she is considering. After going through the whole list (all of the different flowers), she can easily recall those that she had tagged–recall them from a Memento. Play the little app and download the source code before going further:
Communicating with HTML and JavaScript
Working HTML and JavaScript into PHP is no great shakes, and most PHP developers probably have done so at one time or another. However, most of the time I find myself creating horrible mixes of code for a one-off use with nothing encapsulated. The goal here is to see how everything in the application can be encapsulated and at the same time communicate. The purpose here is to find a single state variable that is used by HTML, JavaScript and PHP. Further, that state must be available for placing into a Memento object and stored for later use. (This post simply examines one way to encapsulate everything and have them communicate; however, material from this post will be used in developing a Memento example in a future post.) Also, I wanted to import all of the JavaScript and CSS separately. Figure 1 shows the general plan. (The ‘gardner’ folder contains the flower JPEG images.)
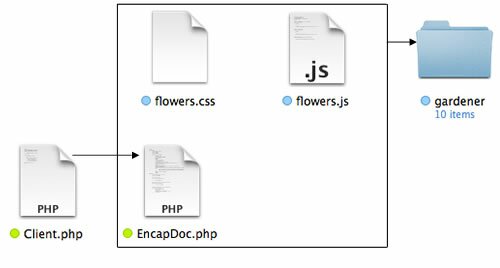
Figure 1: Encapsulating JavaScript, CSS and HTML into EncapDoc PHP class
The CSS is just the stylesheet and it contained no functionality that you often find when it is used in conjunction with jQuery. I needed the JavaScript for clicking through the images. Had I swapped images using PHP I’d probably have to reload an HTML page with every swap and that seem prohibitively expensive. So I wrote the most simple JavaScript swap program I could think of with two functions for swapping and an added JavaScript function to get the initial starting picture (an integer value) passed from PHP. The following JavaScript listings shows how simple the script is:
Continue reading ‘PHP OOP: Encapsulating & Communicating with JavaScript and HTML5’
Recent Comments