Immutable
In his book on Functional Programming in PHP Simon Holywell laments the lack of immutable structures in PHP, and while he suggests some hacks to insure immutability, we can make-do with some different hacks I’ll suggest. (Most of the hacks are mind-hacks–a way of thinking about data.) The idea of having a programming language where all objects are immutable (unchanging) sounds pretty awful. Not only that, it sounds impractical. Take, for example, a Boolean. It has two states; true and false. In functional programming, that means the Boolean variable is mutable, and so it’s out. However, you can have two objects that we can call, Alpha and `Alpha. Alpha is true and `Alpha is false. (The tick mark [`] is the key below the ‘esc’ key on your keyboard.) So instead of changing the state of Alpha from true to false, you change the object from Alpha to `Alpha.
Why would anyone want to do that? It has to do with the concept of referential transparency. In a concrete sense it means that if an object (reference) were replaced by its value, it would not affect the program. Consider the following:
$val=5;
$alpha= function() use ($val) {return $val * $val;};
can be replaced by;
$alpha=25;
Nothing in the program will change if either $alpha variable is used. For a simple example of referential transparency, that’s no great shakes. Besides we lose the value of changing states. However, functional programming eschews the concept of changing states. To quote one functional programmer,
Do not try to change the state; that’s impossible. Instead only try to realize the truth: There is no state.
Again, this looks nuts both conceptually and in the real world. Take, for instance, a thermometer that changes from freezing (32F / 0C) to not freezing (say 50F / 10C). The temperature has changed states! How can anyone say it has not? Or a child changes states into an adult, or a caterpillar changes states to a butterfly?
According to the functional programming model, a freezing temperature is a different object than a non-freezing one; an adult is a different object than a child, and (clearly) a butterfly is a different object than a caterpillar. So, if I say that the thermometer has changed from 32° to 33°, it is not state that has changed, it is a different object. Objects can be as granular as you like, and if you think of atoms arranged to display a ruler, you can move from one atom (object) to the next atom (object) with no state involved at all.
The State Design Pattern: Wasn’t it Immutable All Along?
The State design pattern would seem to be the polar opposite of functional programming. However, if we examine it closely, we can re-conceptualize it as object swapping. Take a simple two-state example: a light going on and off. There’s a light-on object and a light-off object. The design is the same, but we think about it in different ways. Also, the individual state methods can include nothing but lambda functions or closures. Consider Figure 1. An “on” light JPG and an “off” light JPG can be considered two separate states or two immutable objects.
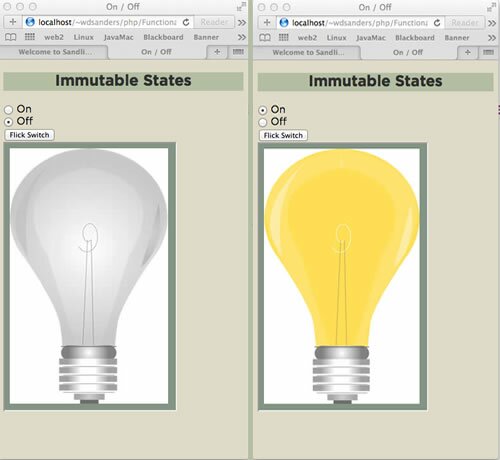
Figure 1: Two States or Two Immutable Objects
To make the State pattern more “immutable-like” the interface has two constants with the URLs for the two different images. To get started, Play the light switch State application and Download the files:
The application uses a simple State design pattern. All requests go through the Context, which keeps track of the current state. However, this implementation fudged a bit because each time the UI calls the Client, it creates a new Context object; so no state is saved, and I had to add a statement to use the called method to set the correct state for switching the light on and off. (Note to self: Get busy on the RESTful API!) Also, I added two constants to the interface (IState) to impose an immutable property in the state implementations. Figure 2 shows the class diagram of the implementation:
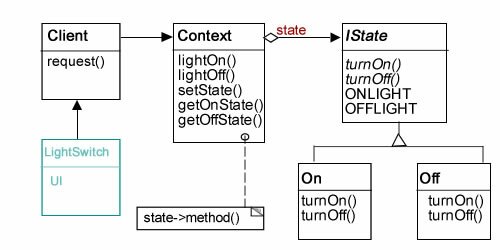
Figure 2: State design pattern implementation
The pattern diagram in Figure 2 provides an overview of the classes and key methods in those classes. The LightSwitch class is just an HTML document wrapped in a PHP class, an it is where a request originates in this model. The other roles you can see in the following outline:
- Client: Get the request from the UI (LightSwitch) and using a Context instance and method, the request is sent to the Context.
- Context: Always the most important participant in a State design pattern, it determines the current state and passes the request to it via the appropriate method based on the request.
- IState: The State interface specifies the required methods and may include constants.
- Concrete States: The On / Off states (IState implementations) return the requested state-as-an-object.
With that overview in mind, you can better understand all of the singular roles of the participants. (Continue to see listings and explanations.)
Continue reading ‘PHP Functional Programming Part II: OOP & Immutable Objects’
Recent Comments