Happy New Year Everyone!
For the last ten weeks I’ve been learning functional programming and Haskell through an edX MOOC offered through Delft University (DelftX) in the Netherlands. (TU Delft is The Netherland’s equivalent to MIT in the US) Check out the YouTube video on the course here. That’s why I haven’t been creating new posts for this blog. Now it’s time to catch up! So, I’ve created a maze game that explores the major principles in design pattern programming using a State design pattern.
Play with a Purpose
This particular maze follows a trail of OOP and Design Pattern principles to the end of the maze. As you find each principle, you will see an image and a statement of the principle. For example, the first part of the maze moves through the S.O.L.I.D. acronym to help you remember five basic OOP principles. When you find the room with the Interface Segregation principle, Figure 1 shows what you will see:
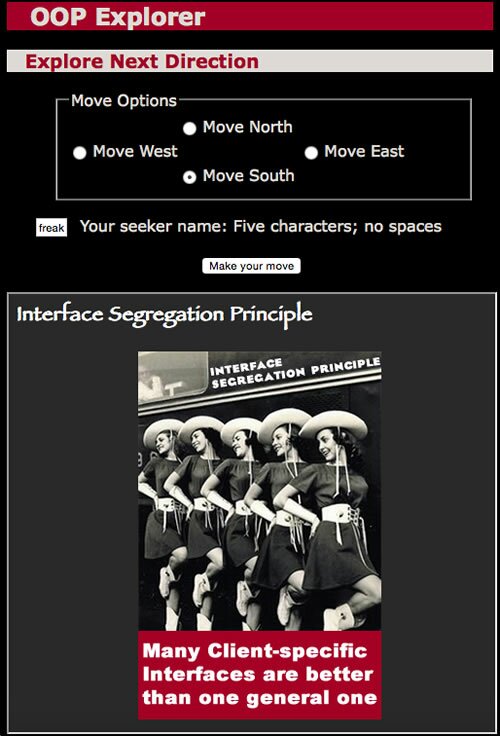
Figure 1: A room in the maze with an OOP principle.
Movement is controlled by a set of four ratio button and a “Make Move” button. Each user must include a unique user name where the moves for the user are stored in a Json file. A State Design Pattern helps not only in creating this maze, but it is a template for any 5 x 5 maze!
Why use a State Pattern on a Maze?
In building a D&D style maze, I started out with a blank sheet of paper and sketched out a 5 x 5 maze shown in Figure 2 (with labels).
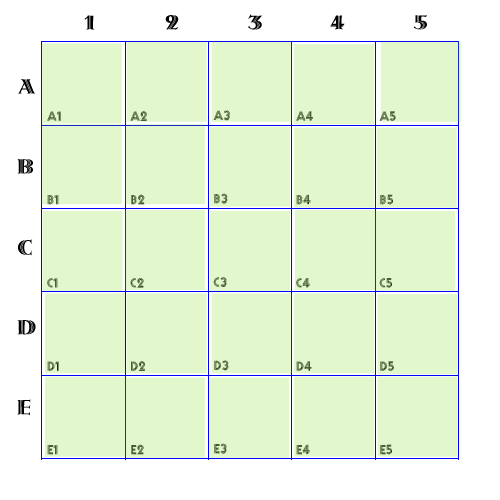
Figure 2: The 5 by 5 Matrix — coordinate values will become class names.
By picturing the matrix as being made up of 25 different states, the reason for using a State design pattern starts to take shape. If each grid square is a state, we can create code that determines what happens to the player who moves into a given state (square).
Adding Start/Finish Points and Trouble
You can decide which states will be the starting and ending states simply by designating them as such. As you can see in Figure 3, the game starts in B1 and ends in D5. The next step is to add back-to-the-start traps. These represent any kind of booby-trap you care to add to make the game interesting. You want to add enough to make the player pay attention but not so many as to make it impossible. Figure 3 shows six sequential traps–game re-start conditions that must be avoided.
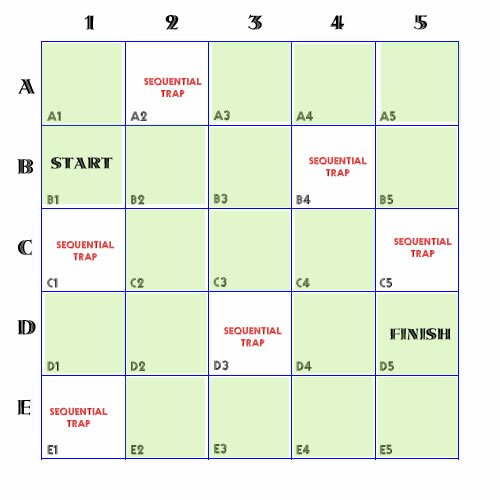
Figure 3: Add start and end states and booby-traps.
In building your maze, keep in mind that for the player, it will seem like a cavern; not the chessboard that you can see. Continue reading ‘The Design Pattern Principle Maze Part 1: A Story in a State Pattern’
Recent Comments