At this point in the Sandlight CMS development process, two design patterns have been employed: 1) Chain of Responsibility as a device checker, and 2) Abstract Factory for making different parts for different devices. As usual, I’ve been posting the results so far on the Sandlight Productions, LLC website. The site itself is the example used in this series. This post is Part IV of the Sandlight CMS series, and if you have not been following the series you can review what has been done so far beginning with Part I.
At this stage, the project is ready to use the Abstract Factory to produce the needed parts and put them together into a page. However, before going ahead and have the factory build the parts I’d like to take an intermediate step and just build a dynamic responsive Web page.
The Dynamic Responsive Web PHPage
The easiest way to proceed is to begin with an HTML5 page, encapsulate the page into a PHP object (class) and add methods. The reason for this step is that the Web site, in addition to being responsive, needs to be dynamic. This means that it needs a way for the administrator to add changes, and in order for this to transpire, the dynamic portion of the page has to include variables in the place of static text, graphics and/or video. Figure 1 shows the general layout envisioned for the page:
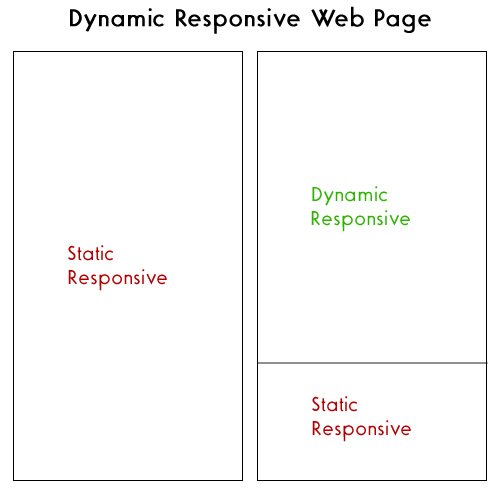
Figure 1: Dynamic Responsive Web Page
Everything about the page must be responsive, but some parts are static and some are dynamic. In the two-column outline in Figure 1, the entire left column is static yet it must be responsive to different devices. In Parts I to III of this series, the pages have been responsive. The next step in making them both responsive and dynamic is to create parts of the page that will be dynamic since they are already set up to be responsive. Before continuing, click the Play button to see the progress so far and the Download button for all of the source code and accompanying files:
Using PHP alone, creating a dynamic page is simple. Just place PHP variables where content goes. This is true for both the pages formatted by CSS3 and jQuery. Importantly, though, all of the Web pages should be encapsulated into PHP classes, using heredoc string variables as has been shown in previous installment of this series.
QR Code: The New Responsive Component
Quick Response Code (QR) is a new necessity for Web sites doing business on the Web and for just about every other kind of site that wants to increase its exposure. Basically, using a QR Code reader in a mobile device (phone or tablet) automatically brings up the link coded in the QR image (a square with some squiggly block images.) That beats trying to to thumb in a URL on a tiny phone keyboard. The following two can be downloaded free (at the time of this writing.):
I’m pretty certain that other smart phones have QR readers; so if you have something other than an iOS or Android operating system on your mobile device, an Internet search for “QR Scanner” will probably be able to locate one for you.
Once you have a QR scanner, you need a coded image, such as the one to your right. Go ahead and scan it to see what happens. It should take you to the Sandlight Productions, LLC home page build on this CMS (updated.) On it you will find several more QR codes for links to sites related to Sandlight. You can get the coded images online at different sites. One site allows you to include your logo and choice of colors is QRCode Monkey. The QR code image in green to your left with the Sandlight logo in the middle does exactly the same thing as the black one. So, if you’d like your QR Code images to keep your graphic designer happy, consider the visual options beyond the default. Figure 2 shows a page being scanned with an iPhone (left) and the linked page in the iPhone (right).
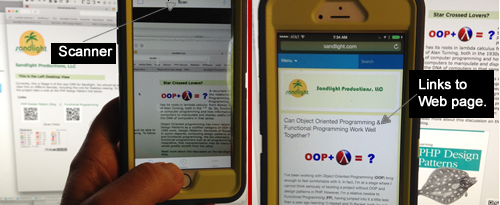
Figure 2: Scanned QR URL uploaded in mobile phone.
Adding QR codes to your site (or your client’s site) is very easy, and you should definitely include it in your dynamic responsive CMS. Let’s now see how the dynamic responsive two-column page is created:
Continue reading ‘Sandlight CMS IV: Dynamic Responsive Web PHPages’
Recent Comments