Solve Once; Use Often
One of the goals, indeed the purpose, of most OOP is to solve a programming problem one time and then re-use it. The idea of re-using code is not so much cutting and pasting code as it is to have a good solution and use the same solution for similar problems. In a larger context, that’s exactly what design patterns do. So far in this CMS series, we have not had to add much to the original DocHere class or to the CSS file. In this step, the only class you will need to change is the Content class developed in in Part II of the PHP CMS Series.
The sample page content has been changed, but all of the new data are from external sources (not unlike the graphics and CSS.) Figure 1 shows the new data in the old page:

Figure 1: All of the data are from external sources of both the PHP files and Web page.
As you can see, everything in the page is in the original style and arrangement. That’s the way it’s supposed to be. All that’s going on is changing data to an existing Web page. The difference in this step is that all of the data are stored in text or graphic files external to the Web page and PHP objects. Everything in this installment is identical to the classes in Part II. All you will need to do is to make some changes in the Content class. The class still uses the same associative array to pass data to the Web page; however, instead of getting its data from literals in the Content class, it gets content from external text files.
Getting Content from the Outside
Before looking into more complex (and robust) ways of adding and/or changing content in a Web page, consider how simple it is using humble text files. Figure 2 shows how the data from an external source becomes the value in a key-value pair in an associative array:
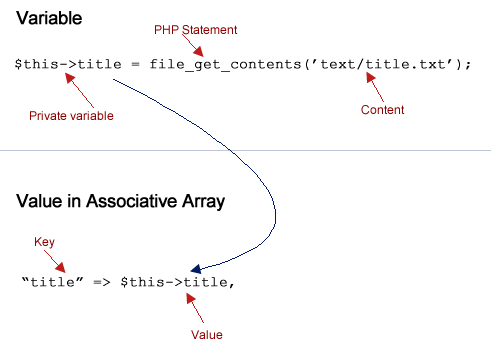
Figure 2: Substituting external text file data for literal value in associative array.
So all that needs to be done with the Content class is to make changes so that it now picks up the data for the Web page from external sources. Actually, every time the Content class has loaded a graphic, it got the data from an external file—a URL. So, all this does is the same thing you’ve done with graphics but with text data.
include_once('PageMaster.php');
class Content extends PageMaster
{
private $title;
private $header1;
private $header2;
private $body1;
private $body2;
private $caption1;
private $caption2;
public function getContent()
{
$this->loadContent();
$this->content = array("css" => "heredoc.css",
"title" => $this->title,
"header1" => $this->header1,
"header2" => $this->header2,
"body1" => $this->body1,
"body2" => $this->body2,
"image1" => "images/bobbed.png",
"image2" => "images/daraculaCar.png",
"caption1" => $this->caption1,
"caption2" => $this->caption2,
);
return $this->content;
}
private function loadContent()
{
$this->title = file_get_contents('text/title.txt');
$this->header1 = file_get_contents('text/header1.txt');
$this->header2 = file_get_contents('text/header2.txt');
$this->body1 = file_get_contents('text/body1.txt');
$this->body2 = file_get_contents('text/body2.txt');
$this->caption1 = file_get_contents('text/caption1.txt');
$this->caption2 = file_get_contents('text/caption2.txt');
}
}
?>
|
All of the content for the page must be placed in text files with the appropriate names. Simply writing what you want to appear in the Web page will do the trick. You can use HTML tags in the text files as well, but remember that all you want is to add content; not structure. Using the image in Figure 1, you should be able to determine what goes in each text file.
As you can see very little had to be done to change the Content class so that it works with external data instead of literals assigned to associative array elements. Eventually, this project will lead to using a database, but for now, each step is one at a time.
Adding a Class for Loading Data
It would be pretty easy to make a LoadContent class from the loadContent() method. Then there would be an object to have the data ready for content use by the Web page and a separate one to load the data used for content. In Part IV, the CMS looks at a separate data loading class and what advantages it would have.
Copyright © 2013 William Sanders. All Rights Reserved.
Any ETA on part IV? I love these guides.
Part IV is this coming week. Sometime after Tuesday.